Patterns
Patterns are what emerge when using the foundation elements together with basic objects like buttons and alerts, more complex Javascript components from Bootstrap like tooltips and dropdowns, and AJAX components from PrimeFaces like datatables and commandlinks.
Contents:
Breadcrumbs
The breadcrumbs are displayed under the header, and provide a trail of links for users to navigate the hierarchy of containing objects, from file to dataset to Dataverse collection. It utilizes a JSF repeat component to iterate through the breadcrumbs.
<div id="breadcrumbNavBlock" class="container" jsf:rendered="#{true}">
<ui:repeat value="#{page.breadcrumbs}" var="breadcrumb" varStatus="status">
<h:outputText value=" > " styleClass="breadcrumbCarrot" rendered="#{true}"/>
<div class="breadcrumbBlock">
...
</div>
</ui:repeat>
</div>
Tables
Most tables use the DataTable components from PrimeFaces and are styled using the Tables component from Bootstrap.
<p:dataTable id="itemTable" styleClass="headerless-table margin-top" value="#{page.item}" var="item" widgetVar="itemTable">
<p:column>
...
</p:column>
</p:dataTable>
Forms
Forms fulfill various functions across the site, but we try to style them consistently. We use the .form-horizontal
layout, which uses .form-group
to create a grid of rows for the labels and inputs. The consistent style of forms is maintained using the Forms component from Bootstrap. Form elements like the InputText component from PrimeFaces are kept looking clean and consistent across each page.
<div class="form-horizontal">
<div class="form-group">
<label for="userNameEmail" class="col-sm-3 control-label">
#{bundle['user.username']}
</label>
<div class="col-sm-4">
<p:inputText id="userName" styleClass="form-control"></p>
</div>
</div>
</div>
Here are additional form elements that are common across many pages, including required asterisks, icon tooltips, placeholder text, input info message with popover link, and validation error message.
This field supports only certain HTML tags.
<div class="form-group form-col-container col-sm-9 edit-compound-field">
<div class="form-col-container col-sm-12">
<p class="help-block">
<h:outputFormat value="#{bundle.htmlAllowedMsg}" escape="false">
<f:param value="#{bundle.htmlAllowedTags}"/>
</h:outputFormat>
</p>
<label class="control-label" for="metadata_#{subdsf.datasetFieldType.name}">
#{subdsf.datasetFieldType.localeTitle}
<h:outputText styleClass="glyphicon glyphicon-asterisk text-danger" value="" />
<span class="glyphicon glyphicon-question-sign tooltip-icon" data-toggle="tooltip" data-placement="auto right" data-original-title="#{subdsf.datasetFieldType.localeDescription}"></span>
</label>
<div>
<p:inputTextarea value="#{dsfv.valueForEdit}" id="description" tabindex="#{block.index+1}" rows="5" cols="60" styleClass="form-control" />
<div class="alert-danger" jsf:rendered="#{!empty subdsf.validationMessage}">
<strong>#{subdsf.validationMessage}</strong>
</div>
</div>
</div>
</div>
Buttons
There are various types of buttons for various actions, so we have many components to use, including the CommandButton component and CommandLink component from PrimeFaces, as well as the basic JSF Link component and OutputLink component. Those are styled using the Buttons component, Button Groups component and Buttons Dropdowns component from Bootstrap.
Action Buttons
For action buttons on a page, we include an icon and text label.
<div class="btn-group" jsf:rendered="#{true}">
<button type="button" id="editDataSet" class="btn btn-default dropdown-toggle" data-toggle="dropdown">
<span class="glyphicon glyphicon-pencil"/> Edit <span class="caret"></span>
</button>
<ul class="dropdown-menu text-left">
<li>
<h:outputLink> ... </h:outputLink>
</li>
<li class="dropdown-submenu pull-left">
<a tabindex="-1" href="#">Option</a>
<ul class="dropdown-menu">
<li>
<h:link> ... </h:link>
</li>
<li>
<h:link> ... </h:link>
</li>
</ul>
</li>
...
</ul>
</div>
Action Buttons Block
For the main actions on a page, we use a container block to group them together. They use the Bootstrap justified button groups style class .btn-group.btn-group-justified
in order to create a group of buttons that stretch at equal sizes to span the entire width of its parent.
The Bootstrap theme provides a .btn-primary
style class to highlight the primary action for the user. This stronger color provides extra visual weight and identifies the primary action in a set of buttons on the page. In this example button group from the file page, you can see the Download and Explore options are listed together, providing a more scalable solution to configurable options.
<div class="col-xs-4">
<div id="actionButtonBlock">
<div class="btn-group btn-group-justified">
<div class="btn-group">
<button type="button" id="accessFile" class="btn btn-primary btn-access-file dropdown-toggle" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
Access File
</button>
<ul class="dropdown-menu pull-right text-left">
<li class="dropdown-header">
Download Options <span class="glyphicon glyphicon-download-alt"></span>
</li>
...
</ul>
</div>
</div>
<div class="btn-group btn-group-justified">
<div class="btn-group">
<button type="button" id="editFile" class="btn btn-default btn-access btn-edit dropdown-toggle" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
Edit File <span class="caret"></span>
</button>
<ul class="dropdown-menu pull-right text-left">
<li>
...
</li>
</ul>
</div>
</div>
<div class="btn-group btn-group-justified">
<a href="#" onclick="return false;" class="btn btn-default btn-xs btn-contact" aria-label="Contact Dataset Owner" title="Contact Dataset Owner">
Contact Owner
</a>
<a href="#" onclick="return false;" class="btn btn-default btn-xs btn-share" aria-label="Share Dataset" title="Share Dataset">
Share
</a>
</div>
</div>
</div>
Form Buttons
Form buttons typically appear at the bottom of a form, aligned to the left. They do not have icons, just text labels. The primary button is styled differently.
<div class="button-block">
<p:commandButton id="save" styleClass="btn btn-default" value="#{bundle.saveChanges}" action="#{page.save}" update="@form,:messagePanel" />
<p:commandButton id="cancel" styleClass="btn btn-link" value="#{bundle.cancel}" action="#{page.cancel}" process="@this" update="@form">
<p:resetInput target="@form" />
</p:commandButton>
</div>
Icon-Only Buttons
There are a few places where we use icon-only buttons with no text label. For these buttons, we do utilize tooltips that display on hover, containing a text label.
We use the style class .no-text
with the .glyphicon
class to fix spacing issues from margins and padding applied to buttons with text labels.
<p:commandLink styleClass="btn btn-default btn-sm bootstrap-button-tooltip" title="#{bundle.add}" actionListener="#{Page.add(valCount.index + 1)}">
<h:outputText styleClass="glyphicon glyphicon-plus no-text"/>
</p:commandLink>
<p:commandLink styleClass="btn btn-default btn-sm bootstrap-button-tooltip" title="#{bundle.delete}" actionListener="#{Page.remove(valCount.index)}">
<h:outputText styleClass="glyphicon glyphicon-minus no-text"/>
</p:commandLink>
Another variation of icon-only buttons uses the .btn-link
style class from Bootstrap, styling it more like a link while maintaining button behavior. The button group provides space for up to three buttons for a file in the table, and if there are more than three action button, they utilize the “kebab” More Options button dropdown with the .glyphicon-option-vertical
icon.
<div class="btn-group" role="group" aria-label="#{bundle['file.actionsBlock']}">
<ui:fragment rendered="#{true}">
<a class="btn-preview btn btn-link bootstrap-button-tooltip" title="#{bundle.preview}"
href="#{widgetWrapper.wrapURL('/file.xhtml?'.concat(...)}">
<span class="glyphicon glyphicon-eye-open"/><span class="sr-only">#{bundle.preview}</span>
</a>
</ui:fragment>
<p:commandLink rendered="#{true}" styleClass="btn-download btn btn-link bootstrap-button-tooltip"
title="#{bundle.download}"
disabled="#{locked ? 'disabled' : ''}"
process="@this" update="@widgetVar(popup)" oncomplete="PF('popup').show();">
<f:actionListener binding="#{pageBean.function()}" />
<f:actionListener binding="#{pageBean.function()}" />
<span class="glyphicon glyphicon-download-alt"/><span class="sr-only">#{bundle.download}</span>
</p:commandLink>
<div class="btn-group" jsf:rendered="#{true}">
<a class="btn-explore btn btn-link bootstrap-button-tooltip"
title="#{bundle.explore}" id="exploreBtn" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false" data-original-title="#{bundle.explore}">
<span class="glyphicon glyphicon-equalizer"/><span class="sr-only">#{bundle.explore}</span><span class="caret"></span>
</a>
<ul class="dropdown-menu multi-level pull-right text-left" aria-labelledby="exploreBtn">
<ui:repeat var="tool" value="#{exploreTools}">
<li>
<p:commandLink styleClass="#{locked ? 'disabled' : ''}"
disabled="#{locked ? 'disabled' : ''}"
action="#{pageBean.function()}">
#{tool.getDisplayNameLang()}
</p:commandLink>
</li>
</ui:repeat>
</ul>
</div>
<div class="btn-group" jsf:rendered="#{true}">
<a class="btn-options btn btn-link bootstrap-button-tooltip"
id="optionsBtn" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false" title="#{bundle.moreOptions}" data-original-title="#{bundle.moreOptions}">
<span class="glyphicon glyphicon-option-vertical"/><span class="sr-only">#{bundle.moreOptions}</span><span class="caret"></span>
</a>
<ul class="dropdown-menu multi-level pull-right text-left" aria-labelledby="optionsBtn">
<ui:repeat var="options" value="#{fileOptions}">
<li>
<p:commandLink styleClass="#{locked ? 'disabled' : ''}"
disabled="#{locked ? 'disabled' : ''}"
action="#{pageBean.function()}">
...
</p:commandLink>
</li>
</ui:repeat>
</ul>
</div>
</div>
Pagination
We use the Pagination component from Bootstrap for paging through search results.
<ul class="pagination">
<li class="#{include.page == '1' ? 'disabled' : ''}">
<h:outputLink value="#{page.page}">
<h:outputText value="«"/>
...
</h:outputLink>
</li>
<li class="#{include.page == '1' ? 'disabled' : ''}">
<h:outputLink value="#{page.page}">
<h:outputText value="< #{bundle.previous}"/>
...
</h:outputLink>
</li>
...
<li class="#{include.page == include.totalPages ? 'disabled' : ''}">
<h:outputLink value="#{page.page}">
<h:outputText value="#{bundle.next} >"/>
...
</h:outputLink>
</li>
<li class="#{include.page == include.totalPages ? 'disabled' : ''}">
<h:outputLink value="#{page.page}">
<h:outputText value="»"/>
...
</h:outputLink>
</li>
</ul>
Labels
The Labels component from Bootstrap is used for publication status (DRAFT, In Review, Unpublished, Deaccessioned), and Dataset version, as well as Tabular Data Tags (Survey, Time Series, Panel, Event, Genomics, Network, Geospatial).
<span class="label label-default">Version 2.0</span>
<span class="label label-primary">DRAFT</span>
<span class="label label-success">In Review</span>
<span class="label label-info">Geospatial</span>
<span class="label label-warning">Unpublished</span>
<span class="label label-danger">Deaccessioned</span>
Alerts
For our help/information, success, warning, and error message blocks we use a custom built UI component based on the Alerts component from Bootstrap.
<div class="alert alert-success" role="alert">...</div>
<div class="alert alert-info" role="alert">...</div>
<div class="alert alert-warning" role="alert">...</div>
<div class="alert alert-danger" role="alert">...</div>
Message Classes
Style classes can be added to p
, div
, span
and other elements to add emphasis to inline message blocks.
Select Dataverse collections to feature on the homepage of this Dataverse collection.
Search query returned 1,000 datasets!
Permissions with an asterisk icon indicate actions that can be performed by users not logged into the Dataverse installation.
Are you sure you want to remove all roles for user dataverseUser?
Please select two versions to view the differences.
<p class="help-block">
<span class="text-muted">...</span>
</p>
<p class="help-block">
<span class="glyphicon glyphicon-ok-sign text-success"></span> <span class="text-success">...</span>
</p>
<p class="help-block">
<span class="glyphicon glyphicon-asterisk text-info"></span> <span class="text-info">...</span>
</p>
<p class="help-block">
<span class="glyphicon glyphicon-warning-sign text-warning"></span> <span class="text-warning">...</span>
</p>
<p class="help-block">
<span class="glyphicon glyphicon-exclamation-sign text-danger"></span> <span class="text-danger">...</span>
</p>
Images
For images, we use the GraphicImage component from PrimeFaces, or the basic JSF GraphicImage component.
To display images in a responsive way, they are styled with .img-responsive
, an Images CSS class from Bootstrap.
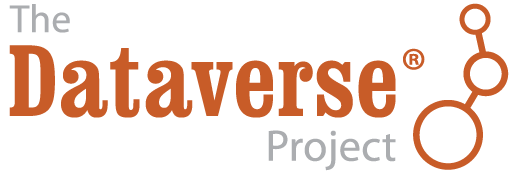
<p:graphicImage styleClass="img-responsive" value="#{Page.imageId}?imageThumb=400" />
Panels
The most common of our containers, the Panels component from Bootstrap is used to add a border and padding around sections of content like metadata blocks. Displayed with a header and/or footer, it can also be used with the Collapse plugin from Bootstrap.
<div class="panel panel-default">
<div class="panel-body">
Basic panel example
</div>
</div>
<div class="panel panel-default">
<div data-toggle="collapse" data-target="#panelCollapse0" class="panel-heading">
<span class="text-info">Panel Heading  <span class="glyphicon glyphicon-chevron-up"/></span>
</div>
<div id="panelCollapse0" class="panel-body form-horizontal collapse in">
<div class="form-group">
<label class="col-sm-4 control-label">
Label
</label>
<div class="col-sm-6">
Value
</div>
</div>
</div>
</div>
Tabs
Tabs are used to provide content panes on a page that allow the user to view different sections of content without navigating to a different page.
We use the TabView component from PrimeFaces, which is styled using the Tab component from Bootstrap.
<p:tabView id="tabView" widgetVar="content" activeIndex="#{Page.selectedTabIndex}">
<p:ajax event="tabChange" listener="#{Page.tabChanged}" update="@this" />
<p:tab id="dataTab" title="#{bundle.files}">
...
</p:tab>
...
</p:tabView>
Modals
Modals are dialog prompts that act as popup overlays, but don’t create a new browser window. We use them for confirmation on a delete to make sure the user is aware of the consequences of their actions. We also use them to allow users to execute simple actions on a page without requiring them to navigate to and from a separate page.
Buttons usually provide the UI prompt. A user clicks the button, which then opens a Dialog component or Confirm Dialog component from PrimeFaces that displays the modal with the necessary information and actions to take.
The modal is styled using the Modal component from Bootstrap, for a popup window that prompts a user for information, with overlay and a backdrop, then header, content, and buttons. We can use style classes from Bootstrap for large (.bs-example-modal-lg
) and small (.bs-example-modal-sm
) width options.
<!-- Large modal -->
<button type="button" class="btn btn-primary" data-toggle="modal" data-target=".bs-example-modal-lg">Large modal</button>
<div class="modal bs-example-modal-lg" tabindex="-1" role="dialog" aria-labelledby="myLargeModalLabel">
<div class="modal-dialog modal-lg" role="document">
<div class="modal-content">
...
</div>
</div>
</div>